You can list all indexes of a table, along with the field names in Laravel. It’s very quick and easy with DB Facade and Laravel Collection.
Here is the code you’ll need. Right, just a single, chained statement! 😉
use Illuminate\Support\Facades\DB;
collect(DB::select("SHOW INDEXES FROM products"))
->mapToGroups(fn($row) => [$row->Key_name => $row->Column_name])
->toArray();
The output will be something like the following.
[
"PRIMARY" => [
"id",
],
"availability_with_price" => [
"price",
"in_stock",
],
]
It’s showing all the indexes as an array. Using index names as array keys and an array of fields as the values (as some indexes may contain multiple fields).
How did it work?
We have used basic MySQL query SHOW INDEXES FROM {TableName}
to retrieve the list of indexes and fields. Each row in the result contains a column (Column_name
) and other details of the associated Index, e.g. the index name (Key_name), uniqueness, etc.

Then we’ve taken the result in a collection and used mapToGroups() to transform the result into an array containing only the index name (as key) and associated fields (as fields).
Here is a screenshot of running the sample code snippet (using Tinkerwell).
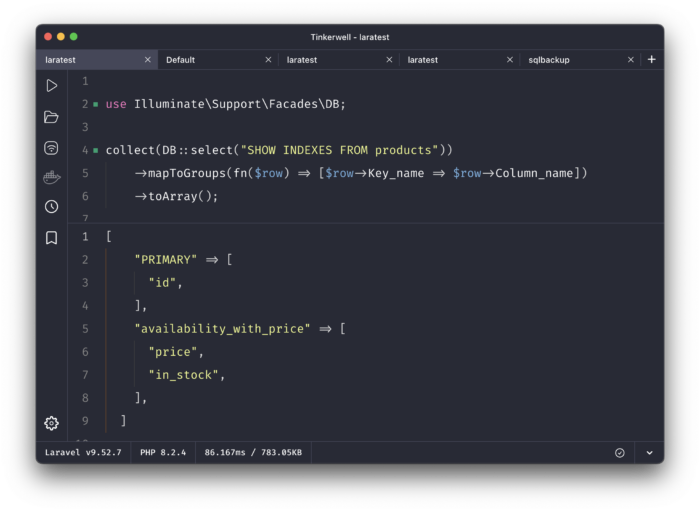
Connect with me on Twitter to stay up-to-date on my latest tips and insights on Laravel and web application architecture.
Happy coding! Build something awesome!